An Ajax call is a mechanism where server side and client side communicates with each other asynchronously and bring data with out browser reloading. An Ajax call is also known as “Half-Duplex”. Because it’s still going through same HTTP process “open-send-close”. Only advantage is, it’s stopping browser reloading for new data.
WordPress has built-in support for AJAX. Every AJAX call should go through admin-ajax.php
file in wp-admin folder. Normally we make a post request to this file with a body parameter action
. this action holds the name of our PHP function which we already have created to get our desired data. We need to hook that PHP function with wp_ajax_{function_name}
. And if we are performing the AJAX call for not login users then we also need to hook up with wp_ajax_nopriv_{function_name}
. admin-ajax.php will listen to our request and call our function_name
function.
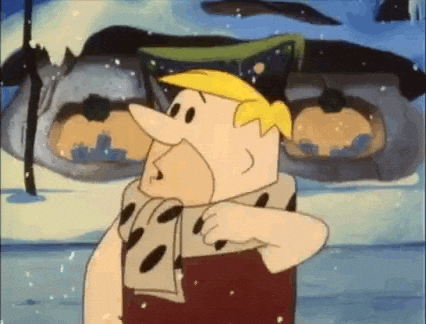
It’s may sound complicated but it’s very easy to achieve in coding. Normally we use jQuery ajax
API to perform the client-side AJAX call. It looks like this:
$.ajax({ type: 'POST', url: 'https://example.com/wp-admin/admin-ajax.php', data: { action: "function_name" }, success: function(response) { console.log(response); }, error: function(error) { console.log(error); } });
Behind the scene, it sends a post request to https://example.com/wp-admin/admin-ajax.php/?action=function_name. And the content-type header is application/x-www-form-urlencoded
. Whatever we define in data
object, goes in the body of the request.
It works fine but we also can do this with modern tools like Fetch API or Axios.
In this article, I will show “how to make an AJAX call with fetch API in WordPress context”.
A Fetch request takes two parameters: URL and options object. For URL we will pass our admin-ajax.php URL and for the options object, we will define method, headers and body.
const url = "https://example.com/wp-admin/admin-ajax.php"; fetch(url, { method:"post", headers: { 'Content-Type': 'application/x-www-form-urlencoded' }, body: "action=function_name" })
It will also send the same post request as we already have done before with jQuery. WordPress highly recommend nonce check so we can stay safe from CSRF attack. We can add our custom nonce in the body also.
fetch(url, { method:"post", headers: { 'Content-Type': 'application/x-www-form-urlencoded' }, body: "action=function_name&security=custom_nonce" }) .then( res => res.json() ) .then( data => console.log(data) ) .catch( error => console.log(error) );
On a side note: The WordPress community also has created a NPM package called API Fetch which is built on top of Fetch API.
Full code Example: https://gist.github.com/AbmSourav/79ccb8cc1ad6641a6aa2dcda24a794de